From :- http://timheuer.com/blog/archive/2008/07/30/format-data-in-silverlight-databinding-valueconverter.aspx
Here i just want to update only the code portion of it
<ListBox x:Name="FeedList">
ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Vertical">
<TextBlock FontFamily="Arial" FontWeight="Bold" Foreground="Red" Text="{Binding Title.Text}" />
<TextBlock FontFamily="Arial" Text="{Binding PublishDate}" />
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
Assume this XAML binds to SyndicationFeed.Items data coming from an RSS feed. If we simply bind it the result would be this:
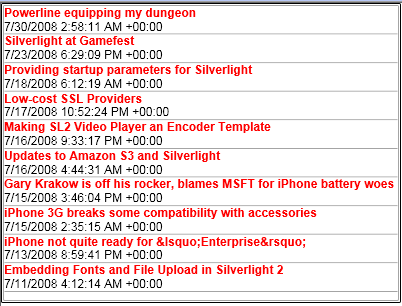
public class Formatter : IValueConverter
{
#region IValueConverter Members
public object Convert(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture)
{
throw new NotImplementedException();
}
public object ConvertBack(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture)
{
throw new NotImplementedException();
}
#endregion
}
public object Convert(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture)
{
if (parameter != null)
{
string formatterString = parameter.ToString();
if (!string.IsNullOrEmpty(formatterString))
{
return string.Format(culture, formatterString, value);
}
}
return value.ToString();
}
xmlns:converter="clr-namespace:TypeConverter_CS"
Finally the code look like this
1: <UserControl x:Class="TypeConverter_CS.Page"
2: xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
3: xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
4: xmlns:converter="clr-namespace:TypeConverter_CS"
5: Width="400" Height="300">
6: <UserControl.Resources>
7: <converter:Formatter x:Key="FormatConverter" />
8: </UserControl.Resources>
9: <Grid x:Name="LayoutRoot" Background="White">
10: <ListBox x:Name="FeedList">
11: <ListBox.ItemTemplate>
12: <DataTemplate>
13: <StackPanel Orientation="Vertical">
14: <TextBlock FontFamily="Arial" FontWeight="Bold" Foreground="Red" Text="{Binding Title.Text}" />
15: <TextBlock FontFamily="Arial" Text="{Binding PublishDate, Converter={StaticResource FormatConverter}, ConverterParameter=\{0:M\}}" />
16: </StackPanel>
17: </DataTemplate>
18: </ListBox.ItemTemplate>
19: </ListBox>
20: </Grid>
21: </UserControl>
Output
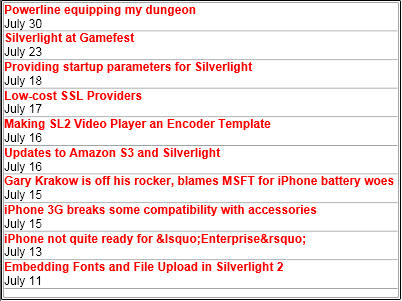